前回まででユーザーの新規登録回りの処理が実装できたので、今回は登録済みユーザー情報の変更処理を実装していきます。
ユーザー情報変更処理の実装
ユーザー情報変更ページの作成
pagesディレクトリにprofile.vueという名前でファイルを作り、適当にユーザー情報変更用のフォームを作ります。こんな感じに作ってみました。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
<template> <form @submit.prevent="updateProfileInformation"> <v-text-field type="text" name="name" id="name" v-model="form.name" label="名前" /> <v-text-field type="text" name="email" id="email" v-model="form.email" label="メールアドレス" /> <v-btn type="submit">変更</v-btn> </form> </template> <script> export default { data() { return { form: { name: this.$auth.user.name, email: this.$auth.user.email, }, }; }, methods: { async updateProfileInformation() { try { await this.$axios.get("sanctum/csrf-cookie"); await this.$axios.put("user/profile-information", this.form); } catch (e) {} }, }, }; </script> |
見た目はこんな感じになります。
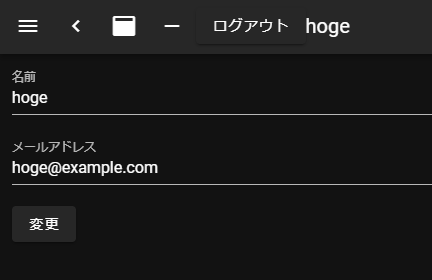
解説
14-15行目:初期値にログイン中のユーザー情報をセットします。
22行目:CSRFの初期化をしています。
23行目:ユーザー情報の更新依頼をputしています。
cors対策
config\cors.phpのpathsに'user/profile-information'を追加します。

ユーザー情報の更新
この状態で更新するとちゃんとリクエストされDBも更新されますがクライアント側の情報は更新されません。更新後にfetchUserメソッドを実行し反映されるようにします。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
<template> <form @submit.prevent="updateProfileInformation"> <v-text-field type="text" name="name" id="name" v-model="form.name" label="名前" /> <v-text-field type="text" name="email" id="email" v-model="form.email" label="メールアドレス" /> <v-btn type="submit">変更</v-btn> </form> </template> <script> export default { data() { return { form: { name: this.$auth.user.name, email: this.$auth.user.email, }, }; }, methods: { async updateProfileInformation() { try { await this.$axios.get("sanctum/csrf-cookie"); await this.$axios.put("user/profile-information", this.form); await this.$auth.fetchUser(); } catch (e) {} }, }, }; </script> |
更新が反映されるようになりました。
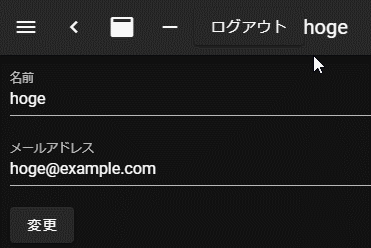
最後に
Laravel側はほとんどいじることなくユーザー情報の更新処理を実装することが出来ました。 次回はページをプロテクトする処理を実装してみようと思います。